Connect
Use Connect to link to your users’ accounts
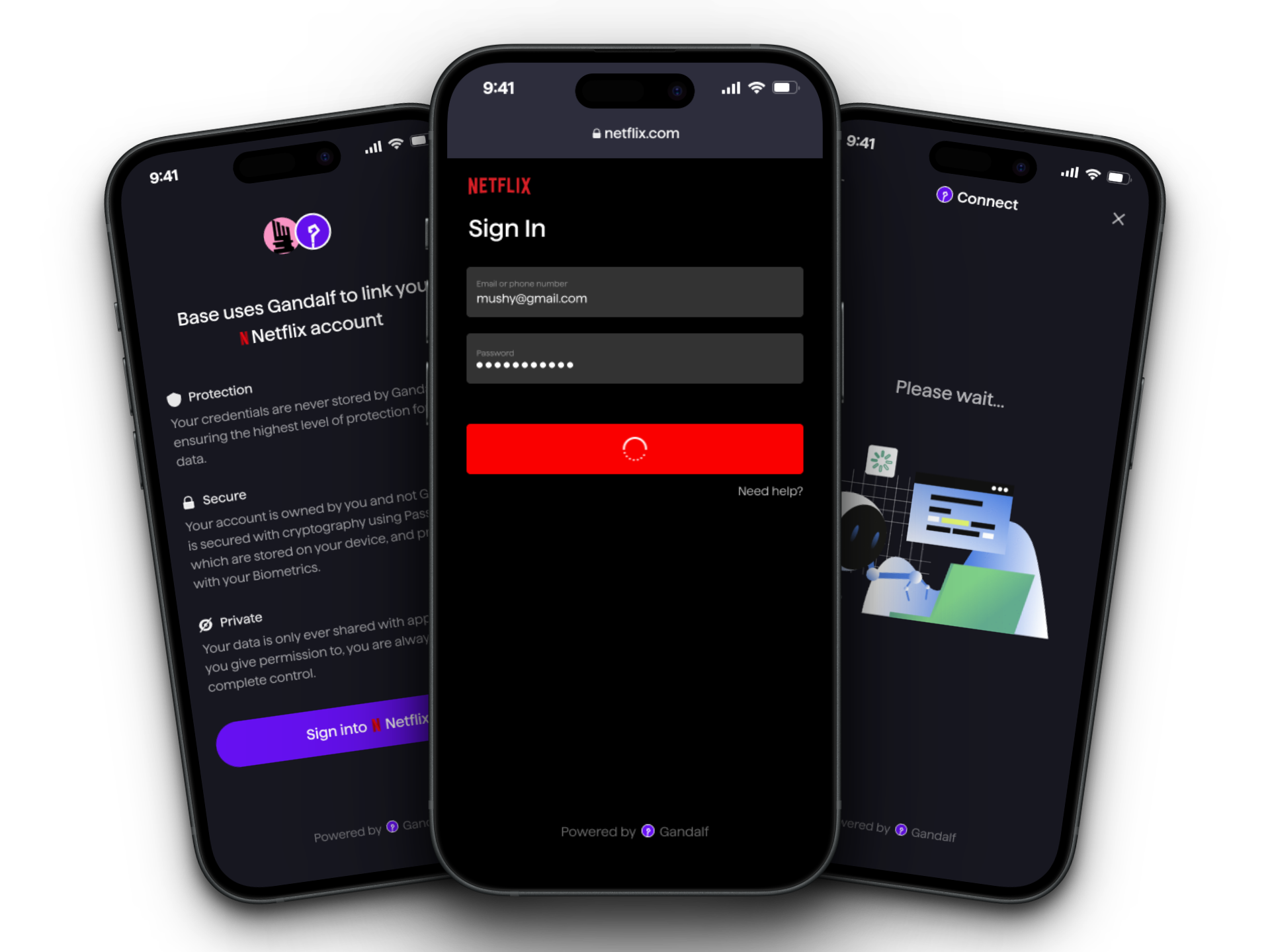
Introduction
Connect is the client-side component that your users will interact with in order to link their accounts to Gandalf and allow you to access their data.
Connect will handle credential validation, multi-factor authentication, and error-handling for every service that Gandalf supports. You can use Connect in any kind of application (web or mobile) - it’s as simple as just adding a link to your app.
Connect is an App Clip. App Clips let users enjoy native app experiences without the need to download a full app. Learn more on Apple’s Developer Website.
To try Connect, check out the Demo.
Connect Flow
The diagram below shows how Connect is used to obtain a dataKey
, which is used server-side to request data.
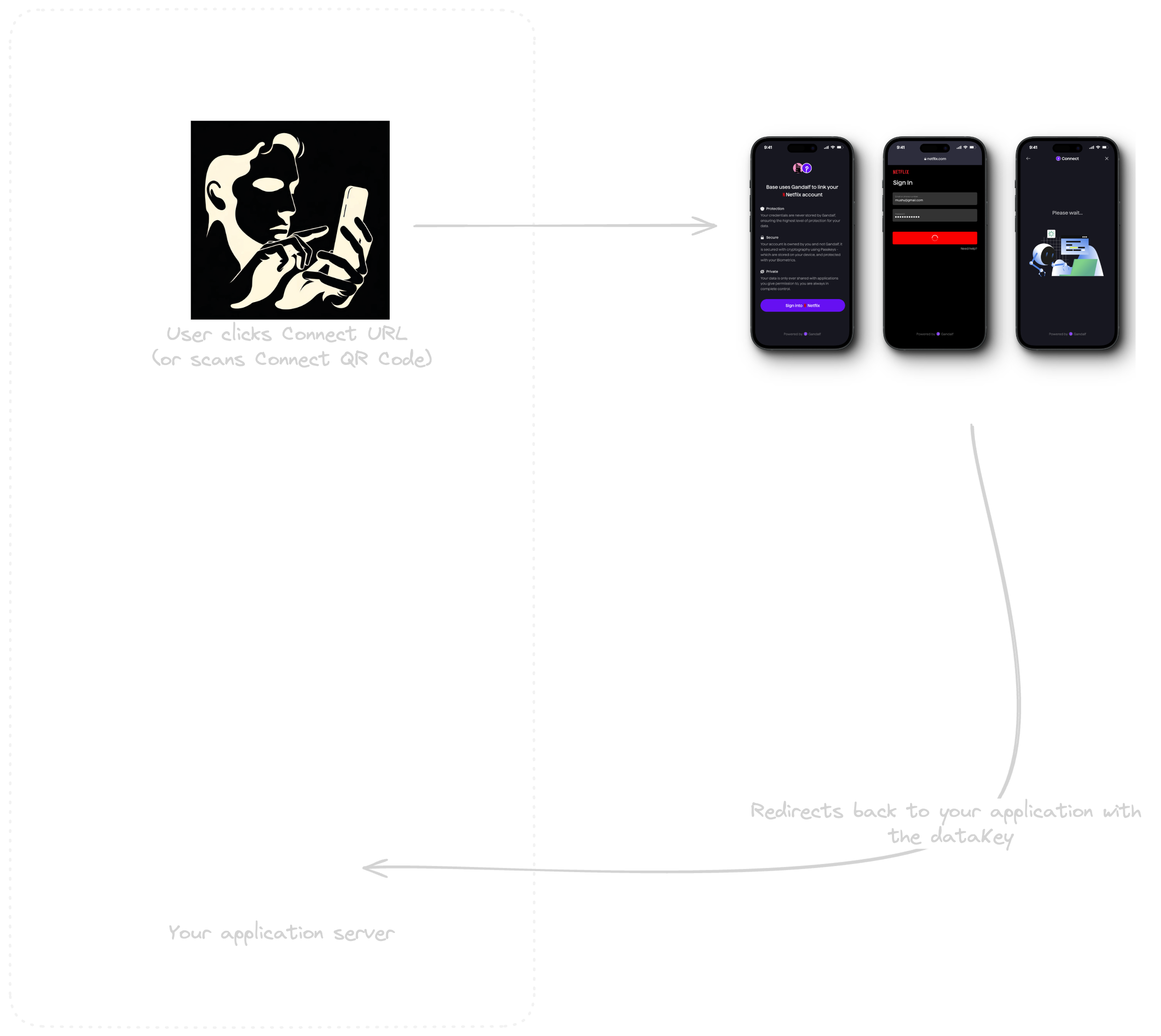
The flow begins when your user wants to link their account/data to your application.
- Open Connect for your users by navigating to the Connect URL. Pass your
publicKey
,redirectUrl
and the service(s) you want to read data from. - After your user is done linking their account(s), Connect will navigate to your
redirectUrl
with a query parameter calleddataKey
. - This
dataKey
will be used to make requests to the Sauron API
Connect URL
Base URL
https://appclip.apple.com/id?p=network.gandalf.connect.Clip
Query Parameters
This is how you specify which services you want to connect to. For example:
{"netflix": true, "playstation": false}
The boolean value lets you determine which service connections are optional or required. Per the example above, your users will be forced to connect their Netflix account, but can choose to skip Playstation.
This specifies where Connect should redirect to after your users have successfully linked their account(s).
Your application’s Public Key.
Connect JavaScript SDK
We provide a JavaScript package to make it a little easier to generate a valid Connect URL or QR Code. The Connect helper handles parameter validations automagically!
Install the connect library
npm i @gandalf-network/connect
Usage
Import the library
// Typescript && ESModules
import Connect from '@gandalf-network/connect'
// CommonJS
const Connect = require("@gandalf-network/connect");
Connect
// Initialize Connect
const connect = new Connect({
publicKey: 'your_public_key_here',
redirectURL: 'https://yourapp.com/connect-success',
services: {'netflix': true, 'playstation': false}
})
try {
// Generate the Connect URL
const connectUrl = await connect.generateURL()
// Use the URL as needed
console.log('Generated Connect URL:', connectUrl)
// If you want to display a QR Code instead:
const qrCodeDataUrl = await connect.generateQRCode()
} catch (error) {
// handle error
}
Display the QR code using the qrCodeDataUrl
<img src={{qrCodeDataUrl}} alt="Connect QR Code"/>